# Set up chat language localization
# What is localization in the RWC?
In the RWC, localization is the translation of system-generated texts to a particular language. It serves to create a more familiar and comfortable environment for international chat users.
TIP
Note, localization only affects the language of the texts generated by the system. The content that you create in the Steps can't be translated. For example, localization does not affect the text of the message you add in any Step.
# Supported languages and locales
The RWC supports the following:
Languages | Language locales |
---|---|
Dutch | nl |
English | en |
French | fr |
German | de |
Polish | pl |
Romanian | rm |
Spanish | es |
Ukrainian | uk |
# How to set up chat localization
- In the Flow, click the Wait for Chat (RWC) Step.
- In the Details tab on the right, scroll down to the Look and feel section, and find the Chat settings subsection.
- In the Chat language dropdown, choose either Auto-detect or any language from the list.
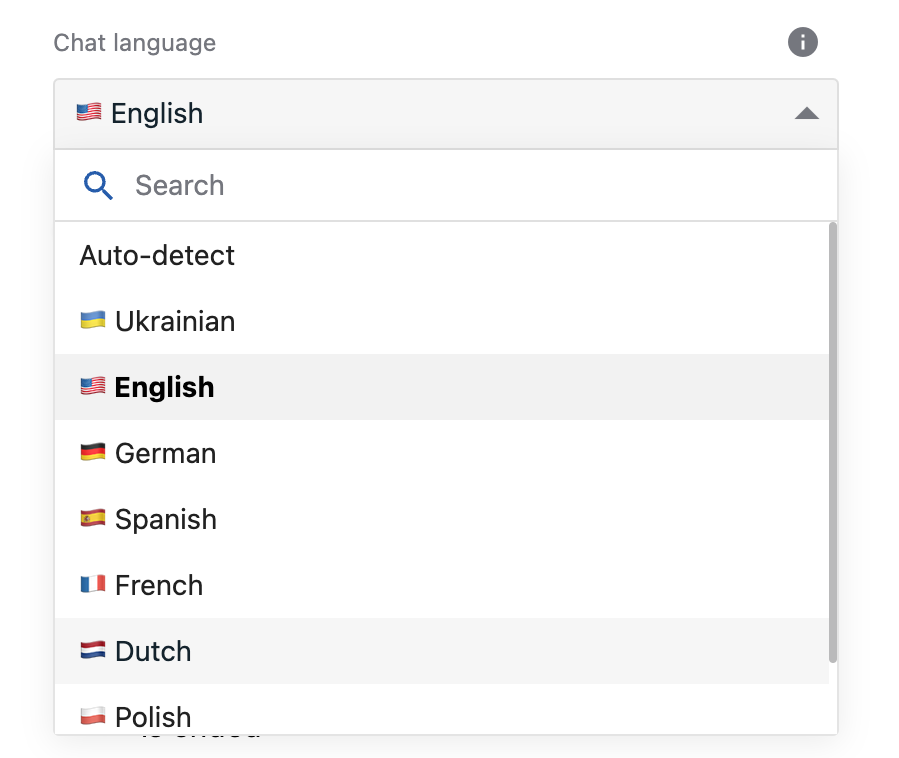
- If you chose Auto-detect, the chat system automatically detects the locale of the user browser and shows system-generated texts in the corresponding language. However, if the system detects a language locale that is not supported by the RWC, system-generated texts are displayed in English.
- Save & Activate the Flow.
# Merge field for chat language
In the Wait for Chat Step, the Chat language setting determines the value of a language
Merge field (opens new window). It can take the following values:
- if in the Chat language, you set a particular language, for example, Ukrainian
{
"language": "uk"
}
- if in the Chat language, you set Auto-detect and it detects a user's browser localization, for example, Ukrainian
{
"language": "uk_UA"
}
- if in the Chat language, you set Auto-detect and it does not detect a user's browser localization that is supported by RWC, the default value gets stored
{
"language": "en_US"
}
# Сode examples to build a simple language selector for a user in the chat
When you build your RWC solution, you can write code to provide chat users with a language selector on the client and let them switch a language dynamically in the chat.
The RWC has the code of its locale service localeService
. Its logic is executed on the frontend. To interact with it, write the related code in the JavaScript or HTML fields of the RWC Steps for it to be executed on the frontend too. For example, write code in one of the following places:
- the HTML field in the Manage Content Area (RWC) Step template
- the JavaScript field in the Set up Global Actions (RWC) Step template
- the JavaScript field in the Custom template user input component of the Request Response (RWC) Step template
- the HTML template field when the Extend page head by custom template toggle is on in the Advanced settings of the Wait for Chat (RWC) Step template
You can use the following commands to interact with the locale service localeService
:
window.localeService.loadLocale('uk') // Call this function and pass the locale as an argument to load a specific language in the chat.
window.localeService.loadUserLocale() // It loads the user locale autodetected in the browser. It gets executed when the chat page is opened.
window.localeService.getSupportedLocales() // It returns an up-to-date list of locales supported by the RWC.
When you execute window.localeService.getSupportedLocales()
, the function returns an array of supported locales. The structure of an item in the array is the following:
{
localeName: 'German', // Name of the locale in English.
originalName: 'Deutsch', // Name of the locale in its language.
locale: 'de', // Short code of the locale.
emoji: '🇩🇪'
}
# Example: Create a simple language selector for chat users
The following code sample retrieves the list of supported languages and displays them in a custom language selector using the getSupportedLocales()
function. As a result, in the chat, the selector dropdown menu displays the supported languages, so it allows the users to change the chat's language on the fly.
const supportedLanguages = window.localeService.getSupportedLocales();
const languageSelector = document.createElement('select');
supportedLanguages.forEach((language) => {
const languageOption = document.createElement('option');
languageOption.value = language.locale;
languageOption.textContent = `${language.localeName} (${language.originalName})`;
languageSelector.appendChild(languageOption);
});
languageSelector.addEventListener('change', (e) => {
window.localeService.loadLocale(e.target.value);
});
document.body.appendChild(languageSelector);
WARNING
You have added the language selector to the chat client. During the conversation, the chat user changes the language in the selector. The system-generated texts in the said messages get translated dynamically.
Note, in this case, dynamic translation will not apply to the following:
- The system message the user gets when they reopen the chat.
- The system message the user gets once the conversation has ended.
Once the user receives these messages, the system determines their language and won't translate them later.